There must be data and an algorithm that operates on that data
In CSV the data is separated by commas, the first line is the title.
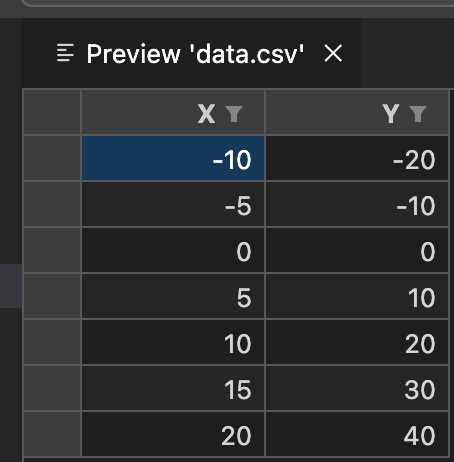
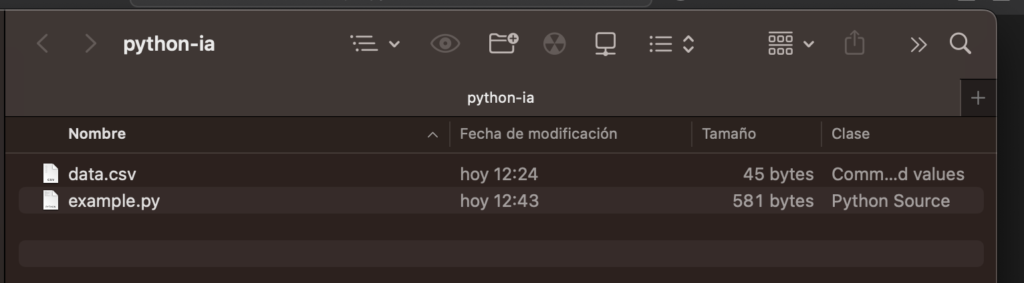
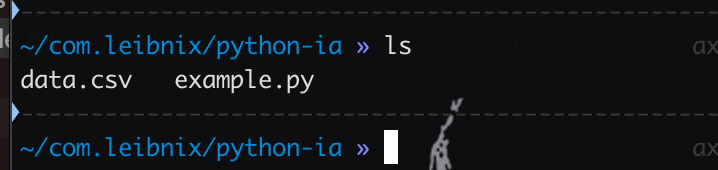
takes 1 or more minutes:

you must use the pip or pip3 dependency manager
pip install pandas
or
pip3 install pandas
If you understand Python, you know what I mean.
If you don’t understand, you must learn Python first.
the code:
import pandas as pd
import seaborn as sb
from sklearn.linear_model import LinearRegression
data = pd.read_csv('data.csv')
sb.scatterplot(x='x', y='y', data=data, hue='y', palette='coolwarm')
x_processed = data['x'].values.reshape(-1,1)
y_processed = data['y'].values.reshape(-1,1)
model = LinearRegression()
# training
model.fit(x_processed, y_processed)
worth = 1
prediction = model.predict([[worth]])
print(f"The prediction for {worth} is {prediction}")
# know if the model is well trained, 1.0 indicates that it is perfect
print(model.score(x_processed, y_processed))
ZsSSA sGOFbJO AZx KTboPh JqQ bISLeo