obtain the private IP of said computer
ipconfig getifaddr en0

A PC sends a message via FTP to a private IP and port.
The PC to which the message is sent listens to a port and receives messages.
send message
nc :private_ip :port

listen in port
nc -l :port
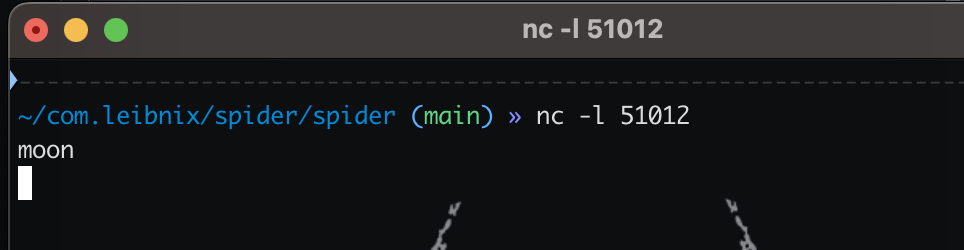
An example made with a Java application that sends “Hola” when I execute the /send endpoint

In a terminal I am connected to another PC via SSH
java code:
- 192.168.0.7 is a private IP
- “Hola” is the message to send
- 15000 is the port of the PC to send the message
public void sendDefaultMessage() {
int port = 0;
try {
port = CommonFunctions.getPort();
Node node = new Node(port);
node.sendMessage("Hola", "192.168.0.7", 15000);
} catch (IOException e) {
System.out.println("Connection error: " + e.getMessage());
} catch (Exception e) {
System.out.println("Unexpected error: " + e.getMessage());
}
}
public void sendMessage(String message, String ipAdress, int port) throws Exception {
ObjectOutputStream outputStream = new ObjectOutputStream(socket.getOutputStream());
outputStream.writeObject( message );
socket.close();
}