It is more than just a binary tree.
Requires that the containing generic class has an ordering relationship defined.
Test coverage: 73%
Maven dependency:
<dependency>
<groupId>com.leibnix</groupId>
<artifactId>binarysearchtree</artifactId>
<version>1.0.0</version>
</dependency>
Here is an article:
https://www.javatpoint.com/binary-search-tree
In short, the elements on the left are less than and those on the right are greater than the value of the central node.
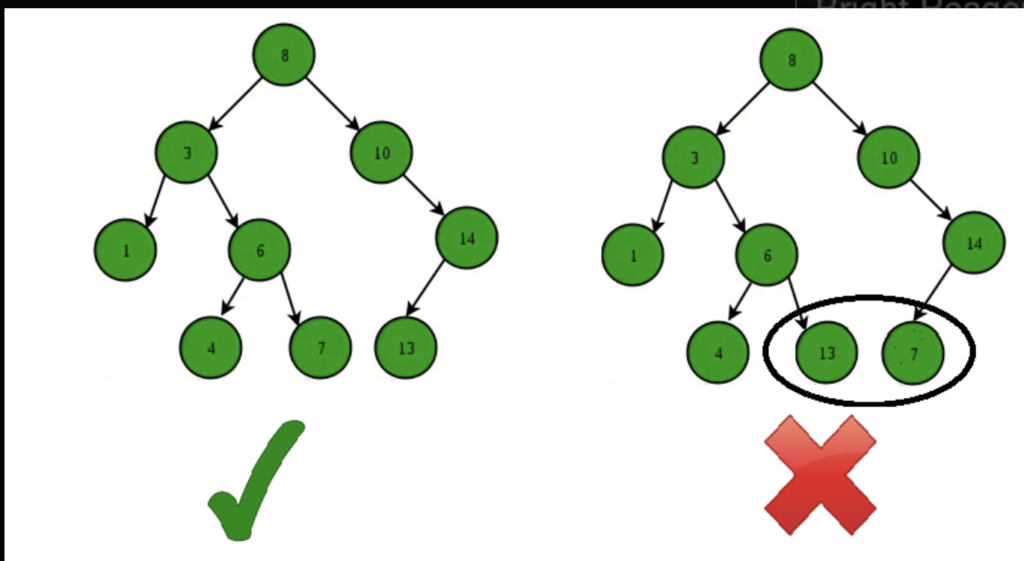
The binary search tree implementation has a MIT license.
The MIT (Massachusetts Institute of Technology) License is a free and open source software license that allows users to use, modify, and distribute the software for free.
Permissions
- Use: You may use the software for any purpose, including personal, commercial, educational, or research use.
- Modification: You may modify the software to suit your needs.
- Distribution: You may distribute the original or modified software to third parties.
Limitations
- Warranty: The software is provided “as is” with no express or implied warranties.
- Liability: The software authors and contributors shall not be liable for any damages or losses in connection with the use of the software.
- Terms
Attribution: You must keep the original copyright notice intact and provide a copy of the MIT License with each copy of the software. - Trademark Usage: You may not use the trademarks of the software authors or contributors without explicit permission.
The MIT License is one of the most popular and widely used software licenses, as it provides great flexibility and freedom to use and modify the software.
You can learn about binary trees here
https://es.wikipedia.org/wiki/%C3%81rbol_binario_de_b%C3%BAsqueda
These articles are in Spanish
As an introduction these articles are fine, but it is not a very difficult data structure to understand if you want to learn more.
BST visual grapher: https://www.cs.usfca.edu/~galles/visualization/BST.html
The BST interface was developed. Are 18 methods, 19 if we count the one that is a comment.
Contains the methods:
void add(T value)
int getHeight()
boolean contains(T value)
void print()
boolean remove(T value)
boolean isEmpty()
boolean isLeaf()
int getQuantity()
void clear()
boolean isFull()
ArrayList preorder()
ArrayList inorder()
ArrayList postorder()
T getRoot()
HashSet asHashSet()
HashMap asHashMap()
T[] toArray()
boolean isBalanced()
boolean isPerfect()
boolean isDegenerate()
boolean isComplete()
boolean isSymmetric()
And BinarySearchTree is its implementation.
In Java you cannot to use a generic class using primitive types like int, double, boolean etc.
Generic classes in Java require the generic type to be a wrapper class like Integer, Double, Boolean, etc., or any other class.
This is because primitive types are not objects and therefore cannot be used as generic types.
However, you can use the Integer wrapper class to create a generic class that behaves similarly to how a primitive int type would.
BinarySearchTree var = new BinarySearchTree<Integer>();
The link to the github repository: