One PC is a server, the other is a client, they communicate using the public IP IPv6.
The example is implemented in Java
Attention: The Java file must have the same name as the class and respect upper and lower case.
First the server must be listening on a port:
java code ServerP2P.java:
import java.net.*;
import java.io.*;
public class ServerP2P {
public static void main(String[] args) {
try {
// Create a server socket on port 15000
ServerSocket serverSocket = new ServerSocket(15000);
System.out.println("Server started on port 15000");
// Accept connections
Socket socket = serverSocket.accept();
System.out.println("Connection established");
// Send and receive data
BufferedReader reader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter writer = new PrintWriter(socket.getOutputStream(), true);
// Send message
writer.println("Hello from server");
// Receive message
String mensaje = reader.readLine();
System.out.println("Message received:" + mensaje);
// Close sockets
socket.close();
serverSocket.close();
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
compile the file
javac ServerP2P.java
then run the code:
java ServerP2P
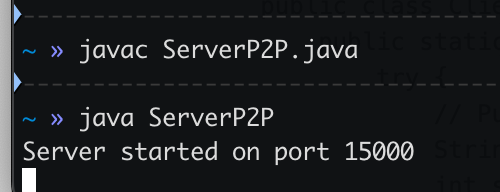
In the following example “2801:812:572:793:743f:8544:f9d4:45d” is the public IPv6 IP, replace it with the public IPv6 IP you want, you can find it on the following pages:
java code of ClientP2P.java:
import java.net.*;
import java.io.*;
public class ClientP2P {
public static void main(String[] args) {
try {
// Public IP address of the server
String ipServer = "2801:812:572:793:743f:8544:f9d4:45d9";
int serverPort = 15000;
// Connect to the server
Socket socket = new Socket(ipServer, serverPort);
System.out.println("Connected to the server");
// Send and receive data
BufferedReader reader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter writer = new PrintWriter(socket.getOutputStream(), true);
// Receive message from the server
String message = reader.readLine();
System.out.println("Message received:" + message);
// Send message to the server
writer.println("Hello from client");
// Close socket
socket.close();
} catch (UnknownHostException e) {
System.out.println("Error: Server not found");
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
compile the code in other PC:
javac ClientP2P.java
run the compiled code:
java ClientP2P
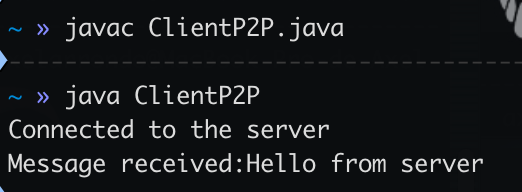
all working together:
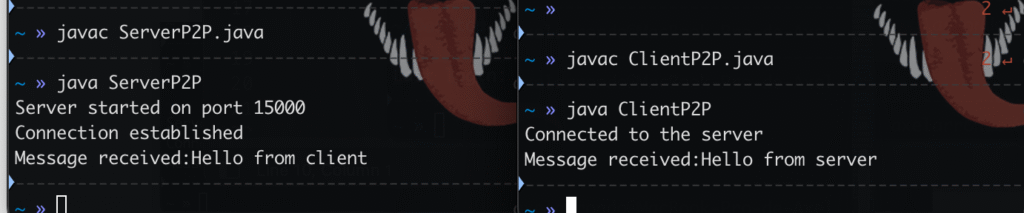
(DDNS) dynamic url mapped to public ip ipv6
tcp.from-ar.com is mapped to an IPv4 and IPv6 with dyn. (works only with IPv6)
java code:
import java.net.*;
import java.io.*;
public class Client {
public static void main(String[] args) {
try {
String domain = "tcp.from-ar.com";
int serverPort = 12000;
//Resolve domain name to IP address
// Performs domain name resolution (DNS) to
// obtain the IP address associated with the domain name tcp.from-ar.com.
InetAddress[] addresses = InetAddress.getAllByName(domain);
InetAddress iPv6Address = null;
for (InetAddress address : addresses) {
if (address instanceof Inet6Address) {
System.out.println("IPv6: " + address.getHostAddress());
iPv6Address = address;
break;
}
}
// Create a socket that connects to the server
Socket socket = new Socket();
socket.setSoTimeout(30000); // 30 seconds
socket.connect( new InetSocketAddress(iPv6Address, serverPort) );
System.out.println("Connected to the server");
// Send data to the server
PrintWriter writer = new PrintWriter(socket.getOutputStream(), true);
writer.println("Hello, server!");
// Close the socket
socket.close();
} catch (UnknownHostException e) {
System.out.println("The server could not be found");
} catch (IOException e) {
System.out.println("I/O Error: " + e.getMessage());
e.printStackTrace();
}
}
}
change tcp.from-ar.com for the real DDNS
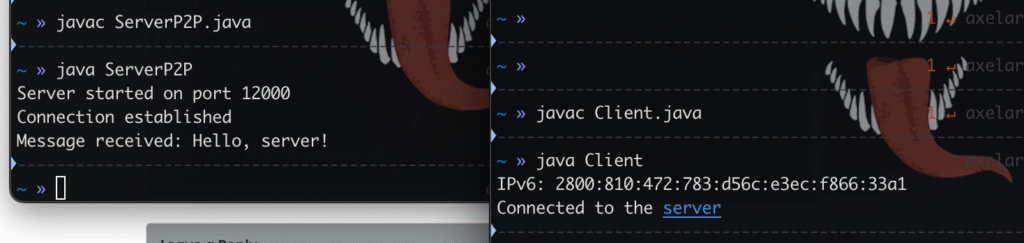
Economy car hire with great terms
toyota vitz rent a car https://www.rent-car-airport.com/our-cars/?view=cardetails&carid=8 .
Дизайнерская мебель для тех, кто ценит красоту и качество.
Мебель премиум-класса Мебель премиум-класса .
Son Sand 30EURo live https://sexdolls.com
Appliance repair: tips and recommendations, for the purpose ofensure the correctness ofdecisions.
air conditioner making strange noises http://www.technirepair.com/4-air-conditioner-repair-tips-for-homeowners .
Быстрое SEO для вашего проекта, для увеличения прибыли.
Прогон сайта GSA http://www.kwork.ru/links/41629912/seo-pushka-dlya-sayta-mnogourovnevaya-piramida-ssylok-pod-klyuch .
Advanced Approaches to Construction, that should be adopted.
foundation types for different soils http://rapidlybuild.com/understanding-different-types-of-building-foundations/ .
for the 2025 Market.
when Finalizing the Deal.
fuel efficiency tips for drivers http://www.livelycars.com/the-most-fuel-efficient-cars-of-2024/ .
Building a team for a successful business, important for the start.
how to create a business plan timetobuiseness.com .
Economy class rentals, with minimal requirements.
car hire paphos town neochorion.com .
Испытайте удачу в Dragon Money Казино
dragon money казино зеркало dragon money казино зеркало .
Обзор казино Драгон Мани: выигрыш и бонусы
sweet bonanza обзоры https://casinowild24.com/sweet-bonanza .
Secrets of Long-Term Operation, of industrial machinery, that are useful for everyone.
industrial machine maintenance schedule i-repairing.com .
A Guide to Leasing a House, to find the best option.
electric car rental cost comparison https://www.rentingforholidays.com/understanding-electrical-equipment-repairs-a-basic-guide .
Quality windows and doors from Republic, with excellent features.
Update your home, with Republic windows and doors.
Classic and modern, Republic.
Quality installation.
Best window and door solutions, with Republic.
Update your home, with quality products.
Energy-efficient doors, by Republic.
A choice that will surprise you, from Republic company.
High-quality windows and doors, at affordable prices.
Beautiful and functional.
Perfect solutions for your style, with Republic.
Window and door selection for your home, from Republic company.
Discounts on window and door solutions, from experienced specialists.
Quality windows and doors for your home, with Republic products.
New solutions from Republic, for your comfort.
Choose only the best, with quality guarantee.
Reduce your energy bills, Republic.
Create coziness in your home, with windows and doors.
Experts in windows and doors, by Republic company.
quality windows and doors quality windows and doors .
In Dubai, balloon delivery options are becoming more common. Celebrations are made even more special with the festive touch of balloon deliveries, appealing to many in Dubai.
yacht decoration dubai http://www.bestwedding-video.com/product-category/yacht-decoration/ .
Integrating a natural terpene blend into your everyday life can potentially enhance your well-being. As awareness about the benefits of terpenes continues to grow, more people are seeking these natural solutions.
terpenes for concentration https://www.chinese-shipments.com/ .